Scala tutorial to demonstrate infix custom notation in scala: syntactic sugar
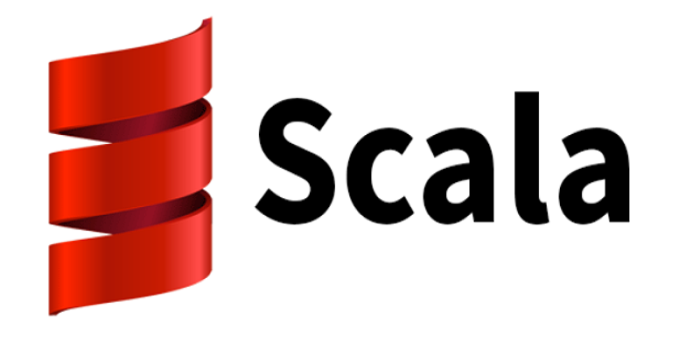
So we know scala mimic certain expressions close to natural language like (obj prints "hello world"), this is possible in scala because all operators are treated as method calls like in expression a + b is internally translated to a.+(b), internally any number or strings is represented as object, most of the primitive object like int , float double has basic methods implemented like + , -, %. We also call this as syntactic sugar..!! syntactic sugar is syntax within a programming language that is designed to make things easier to read or to express. It makes the language "sweeter" for human use.
So now lets create our own operators or override certain operators to define our implementation detail.
Infix notation
If we familiar with any programming language we know what is infix notation are, so in infix notation operators is between two operand i.e 1 + 1
So in scala + is method call 1 is an object. so like wise we shall implement our own infix operator.
Below code implements prints as a infix operator...!!!
object hello {
def prints (input:String) = {
println( s"Hello ${input}")
}
}
so usually we call prints method hello.prints(" world")
but in scala any method having single paramater input can we written as infix notation like hello prints "world"
lets implement + to concat two variable of objects as infix notation
class Hello(val x:String) {
def +(input:Hello):String = {
s"${this.x} ${input.x}"
}
}
object operatorsExample extends App {
val x = new Hello("hi");
val y = new Hello("welcome to scala ");
print(x + y );
}