Factory Pattern In Scala
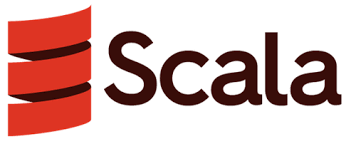
A Factory pattern or Factory method pattern is very common design pattern used in software development,its is classified as creational pattern. which solves the problem of creating the instance of the class without actually specifying exact class need to be created.this pattern is part of Gang of four pattern which was developed keeping SOLID principles in mind. lets consider we have ORM which is capable of connecting to different SQL Database providers like MYSQL or ORACLE based on the configuration we can create required instance during run time to do basic CRUD operations without explicitly specifying object creation. so to make the example easier lets dive into Scala code with very simple example creating an Female or Male Instance of the class.
Step 1: Interface or Abstract class
Interface or abstract class will server as the base class,which abstracts public methods that has to be implemented by the implementing class, Which sort of act like the blue print how the class will behave when consumed by other classes or 3rd part application. so lets create the blue print called Member class specifying the common properties required for Male or Female class. so in Scala we have something called traits which just like an abstract class where we can use this for multiple inheritances so instead of using Abstract or interface i will use trait for providing more loosely coupled behaviour and make the code more flexible and open for updating without changing much code.
private trait Member {
protected val name: String;
protected var mother: Member
protected var father: Member;
protected var spouse: Member;
def _name: String = name
def _mother: Member = mother
def _father: Member = father;
def _mother(mother: Member) = this.mother = mother
def _spouse(spouse: Member) = {
this.spouse = spouse
}
def _father(father: Member) = this.father = father
def _spouse: Member = spouse;
}
Basically i have trait which can store Details of Family member and few implemented abstract method which are common to both gender. but there is only one catch that only female can give birth to child. so lets create or male and female classes having such constrain.
private class Female(protected val name: String,
protected var mother: Member = null,
protected var father: Member = null,
protected var spouse: Member= null,
private var child: List[Member] = List()) extends Member {
def addChildren(child: Member) = {
child._father(this.spouse)
child._mother(this);
this.child ::= child
}
def _children : List[Member] = child;
}
private class Male(protected val name: String,
protected var mother: Member = null,
protected var father: Member = null,
protected var spouse: Member= null) extends Member {
}
So basically we have created the male and female class by extending the behaviour of the Member class. so we shall create Class called Factory, which helps in creating the Instance of the class by come predefined rules during the run time. so deciding clause of creating will be gender as an input.
case object MemberFactory {
def apply(gender: String, name: String): Member = {
gender.toUpperCase match {
case "MALE" => new Male(name);
case "FEMALE" => new Female(name)
case _ => throw new RuntimeException("Invalid Gender");
}
}
}
and lets assume we are creating console application where we get name and gender as input where we have to create the object in the run time. so we shall implement our main method to create the class.
object Solution extends App {
Member person = MemberFactory.apply(args(0),args(1));
}
so if we look at our main class we are not exactly specifying the type of class, instead we are using the base trait as representation. so this will helps to extend our application by having multiple genders in future without having to change much of the code.