Set up App Loading or Progress indicator using dialog box in Flutter
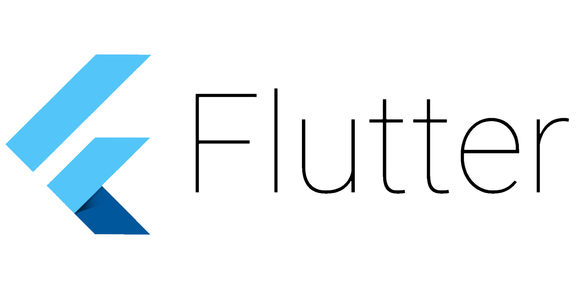
As we know Flutter is Google's portable UI toolkit for crafting beautiful, natively compiled applications for mobile, web. which is rapidly picking up by community these days,while working with hobby project in flutter, i was naively setting state for loading page and resetting the state when request completes, i was redundantly writing the same code again and again in multiple pages, so to overcome this i came up with alternate approach to display the loading Indicator in system dialog without rewriting the major portion of the code. one of the advantage of using dialog is to prevent user interaction and also having native look and feel, this may not be the best looking UX but serves my purpose.
Creating static function to for App loading indicator.
import 'package:flutter/material.dart';
class Dialogs {
static Future<void> showLoadingDialog(
BuildContext context, GlobalKey key) async {
return showDialog<void>(
context: context,
barrierDismissible: false,
builder: (BuildContext context) {
return new WillPopScope(
onWillPop: () async => false,
child: SimpleDialog(
key: key,
backgroundColor: Colors.black54,
children: <Widget>[
Center(
child: Column(children: [
CircularProgressIndicator(),
SizedBox(height: 10,),
Text("Please Wait....",style: TextStyle(color: Colors.blueAccent),)
]),
)
]));
});
}
}
This code display loading icon with the text stating Please wait...
Invoking App Loading Indicator to display the loading when necessary.
....
final GlobalKey<State> _keyLoader = new GlobalKey<State>();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text("Login")),
body: Scaffold(
body: Column(
children: <Widget>[
SizedBox(
width: double.infinity,
height: 50,
child: RaisedButton(
onPressed: () => _handleSubmit(context),
child: Row(children: <Widget>[
Icon(FontAwesomeIcons.google),
Padding(
padding: EdgeInsets.all(1.0),
),
Text("Login")
])))
],
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.end,
),
));
}
Future<void> _handleSubmit(BuildContext context) async {
try {
Dialogs.showLoadingDialog(context, _keyLoader);//invoking login
await serivce.login(user.uid);
Navigator.of(_keyLoader.currentContext,rootNavigator: true).pop();//close the dialoge
Navigator.pushReplacementNamed(context, "/home");
} catch (error) {
print(error);
}
}
}
On any Action like press of a button we can call Dialogs.showLoadingDialog(context, _keyLoader);
and to close dialog Navigator.of(_keyLoader.currentContext,rootNavigator: true).pop();
and make sure to create global key to provide context information for static function to dismiss the dialog.
Screen Shot
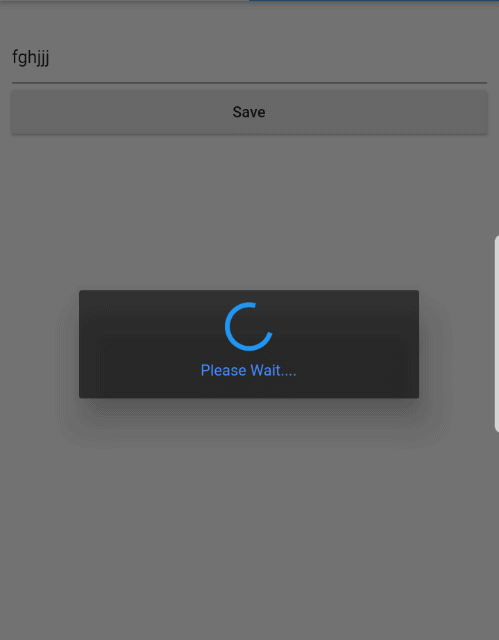