Sending Email Via AWS SES: JAVA Micronaut
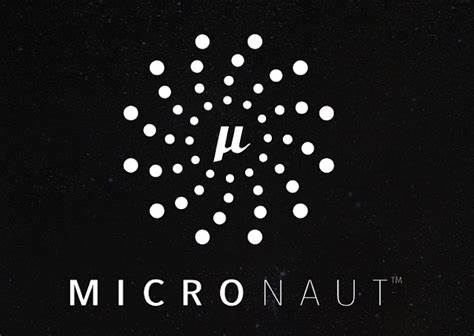
Well we all are familiar with the AWS Cloud services, one such cloud service I often use is SES, its called as Simple Email Service, As name suggest this is used for sending bulk emails. SES is flexible and cost effective, flexible in the sense like can be connected through SMPT or via HTTP API, and this has wide set of SDK available to integrate. So in this article I have used Java as my programming language Micronut as my web framework to demonstrate sending email via http API.
Dependency Required:
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>ses</artifactId>
<version>2.16.22</version>
</dependency>
Creating the SES Client
in this step will be creating SES client which takes AES client id and secret which builds AWS Credentials and return the SES client which will be used for sending the email.
@Singleton
public class SesBean {
@Inject
Environment env;
@Bean
public SesClient getSESClient() {
AwsBasicCredentials credentials = AwsBasicCredentials.create(env.get("aws.clientId", String.class),
env.get("aws.clientSecret", String.class));
SesClient client = SesClient.builder().region(Region.US_EAST_1)
.credentialsProvider(StaticCredentialsProvider.create(credentials)).build();
return client;
}
}
Later we write the adapter class, which implement the sending email method, As we know Adapter patter works like a bridge between two methods ,which works like an wrapper between the SES client.
@Singleton
public class SesAdapter {
@Inject
SesBean ses;
public boolean sendMail(String message,String subject,String toAddress) {
Destination destination = Destination.builder().toAddresses(toAddress).build();
Message SesMessage = Message.builder().body(Body.builder().text(Content.builder().data(subject).build()).build()).subject(Content.builder().data(subject).build()).build();
SendEmailRequest request = SendEmailRequest.builder().destination(destination).message(SesMessage).build();
ses.getSESClient().sendEmail(request);
return true;
}
}
Final step is to invoke the this Adapted method from our controller to trigger the email.
@Controller()
public class EmailController {
@Inject
SesAdapter sesAdapter;
@Post(value = "/mail")
public boolean senMail(@Body EmailTO body) {
sesAdapter.sendMail(body.getBody(), body.getSubject(), body.getToAddress());
return true;
}
}
And now deploy your application as Lambda function, and create the API gateway and send the message.
To know how to create lambda functions with Micronaut use this article:
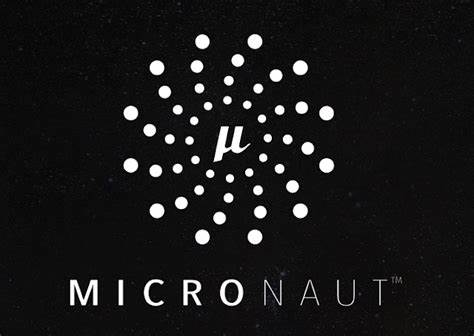