Sending and Receive Message in AWS SQS Using Micronaut Java
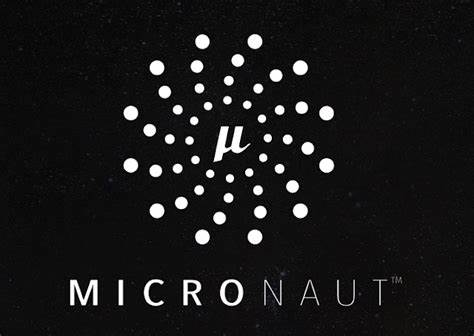
Amazon has SQS is fully managed Message Queuing Service, This is designed to ease the exchanging messages between two systems. And this might helps to decouple the the system. For example to trigger another lambda function which preforms some clean up task in the background or Scrape some data. reason for using the queue will be infinite.
So when it come using the queue is to send the message to the queue programmatically, So this article while guide you to send the message to the Queue from Java,
Step 1 : So Lets add the dependency required.
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>sqs</artifactId>
<version>2.16.14</version>
</dependency>
Step 2: Next Step is to Setup the client which connects with AWS Credentials.
@Singleton
@Introspected
public class SQSBean {
@Inject
Environment env;
@Bean
@Primary
public SqsClient getSqsClient() {
AwsBasicCredentials credentials = AwsBasicCredentials.create(env.get("aws.clientId", String.class),
env.get("aws.clientSecret", String.class));
SqsClient client = SqsClient.builder().region(Region.US_EAST_1)
.credentialsProvider(StaticCredentialsProvider.create(credentials)).build();
return client;
}
}
Step 3 Writing The Adapter which Helps you to Send and receive the message.
@Singleton
public class SqsAdapter {
@Inject
SQSBean sqsClient;
String url = "<Que url>";
public <T> boolean sendMessage(T body) {
ObjectMapper mapper = new ObjectMapper();
try {
sqsClient.getSqsClient().sendMessage(SendMessageRequest.builder().queueUrl(url)
.messageBody(mapper.writeValueAsString(body)).build());
} catch (Exception e) {
e.printStackTrace();
}
return true;
}
public <T> List<T> receiveMessage(Class<T> className) {
ObjectMapper mapper = new ObjectMapper();
try {
ObjectMapper objectMapper = new ObjectMapper();
sqsClient.getSqsClient().receiveMessage(ReceiveMessageRequest.builder().queueUrl(url).build());
ReceiveMessageResponse message = sqsClient.getSqsClient().receiveMessage(ReceiveMessageRequest.builder().queueUrl(url).build());
return message.messages().stream().map(m -> {
try {
return mapper.readValue(m.body(),className);
} catch (JsonProcessingException e) {
return null;
}
}).collect(Collectors.toList());
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
The Adapter helps to send the the messages or receive the message to and fro from the queue.
So lets inject the the SqsAdapter
in controller or service and invoke the method `<>.SendMessage( new User("Test"))` to send some message and `<>.receiveMessage()` to recieve one.