let's start Reusing the Rest Template client instead of repeating the same.
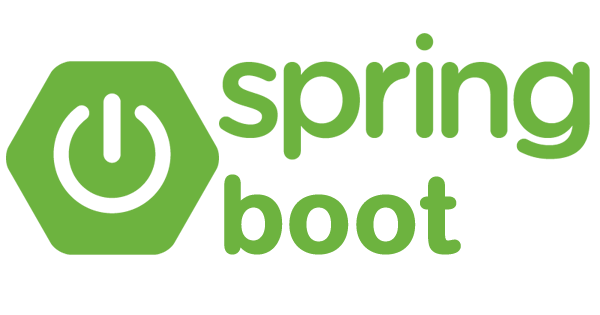
In this article, I am writing the easy and elegant way of writing the rest template, which avoids creating multiple rest clients, which helps in eliminating a lot of boilerplate code and unwanted unit test cases.
FYI: I am using Java Generics to avoid re-writing. So now let dive into the code and, I will explain how does the code work.
public class HttpClient {
private RestTemplate restTemplate;
private ClientHttpRequestFactory factory = new BufferingClientHttpRequestFactory(new SimpleClientHttpRequestFactory());
public HttpClient() {
restTemplate = new RestTemplate(factory);
}
public <R, T> T doRequest(String method, String url, Map<String, String> headers, R body, Class<T> responseType) {
HttpMethod httpMethod = HttpMethod.resolve(method.toUpperCase());
HttpHeaders header = new HttpHeaders();
headers.forEach((String name, String value) -> {
header.add(name, value);
});
HttpEntity<R> httpEntity = new HttpEntity<>(body, header);
return restTemplate.exchange(url, httpMethod, httpEntity, responseType).getBody();
}
}
I will name my class 'HTTPClient' which does the HTTP API call and returns the response from the HTTP request to the caller.
So two-component we require to do HTTP calls are RestTemplate
and ClientHttpRequestFactory
, which I have declared and initiated at the beginning of the class.
And I have written the doRequest
method which does the HTTP call. if you notice <R,T>
at the beginning of the function, this is called as Diamond operator which is instructing the java compiler that the function will receive and return generic objects while calling the function.
just like a normal function, this has a return type of 'T' normally we do return specific DTO in the HTTP client but here I am using the generic object identified as "T" is returned.
In any traditional HTTP call, we do perform specific HTTP operations like "PUT", "POST", "GET", "DELETE" to an URL, providing body and HTTP headers as part of the HTTP call, so the 'request method takes those as parameters which are required, Here the "R" is the generic type which his passed as the body in the request, this generic type can be simple HashMap, string or any DTO, and the last parameter tells the type of return object the caller is expecting.
Now let see how we can call this function. Just Create a Service class and initialize "HTTPClient" and invoke the 'doRequest' method.
Just find the reference below.
public class ExampleService {
HttpClient client = new HttpClient();
public String simpleGETCall() {
Map<String,String> headers = new HashMap<> ();
headers.put(HttpHeaders.ACCEPT,"UTF-*");
headers.put(HttpHeaders.AUTHORIZATION,"<auth token>");
return client.doRequest("GET","https://google.com",headers,null,String.class);;
}
public String simplePOSTCall() {
Map<String,String> headers = new HashMap<> ();
headers.put(HttpHeaders.ACCEPT,"UTF-*");
headers.put(HttpHeaders.AUTHORIZATION,"<auth token>");
String body = "{\"example\":\"exmple\"}";
return client.doRequest("POST","https://google.com",headers,body,String.class);
}
}