JAVA TIP: Removing time and offset for date comparison on Date Object
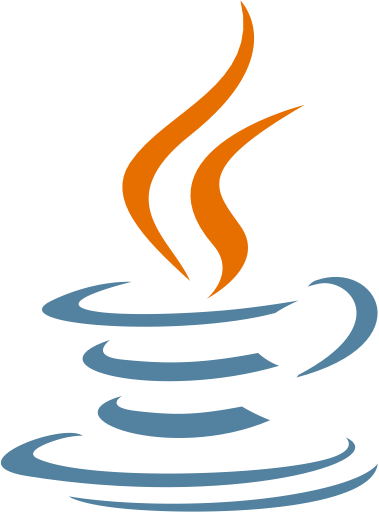
This program helps to remove time append with date object. in certain scenarios we might have stored complete iso string or an timestamp which has time recorded. when we try to compare date using java equals function i.e someDate.equals(withsomeDate) we often end up in mess, so while our intention is to compare only dates ignore time below piece of code set the time to starting of the day, which makes the comparison easier
private Date dateOffsetRemover(Date input) {
try {
SimpleDateFormat dt = new SimpleDateFormat("MM/dd/yyyy hh:mm:ss");
Calendar cal = Calendar.getInstance();
cal.setTime(input);
String tempMonth = "";
String tempDay = "";
if ((cal.get(Calendar.MONTH) + 1) < 10) {
tempMonth = "0" + (cal.get(Calendar.MONTH) + 1);
} else {
tempMonth = "" + (cal.get(Calendar.MONTH) + 1);
}
if (cal.get(Calendar.DAY_OF_MONTH) < 10) {
tempDay = "0" + cal.get(Calendar.DAY_OF_MONTH);
} else {
tempDay = "" + cal.get(Calendar.DAY_OF_MONTH);
}
String sStartdDate = tempMonth + "/" + tempDay + "/" + cal.get(Calendar.YEAR) + " 00:00:00";
Date startDate1 = dt.parse(sStartdDate);
return startDate1;
} catch (Exception e) {
e.printStackTrace();
}
return new Date(input.getTime());
}