Flutter List view: populating the data using List view
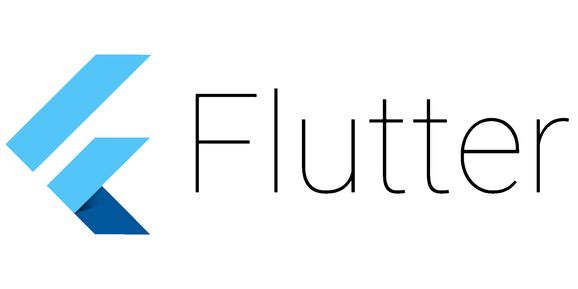
When in Mobile apps list view is crucial, in this article lets see how to build flutter list view using ListView widget and use it in our application, just for information sake Flutter is framework built by google to build multi platform application like Android, Web and IOS. Flutter follows Complete material design Specification. in this tutorial we shall create list view.
Lets begin with creating PODO (Plain old Dart object ) called news.
class News {
String title;
String content;
String description;
String path;
String imagePath;
DateTime publishedAt;
String sourceName;
String sourceId;
News(this.title, this.content, this.description, this.path, this.imagePath,
this.publishedAt, this.sourceName, this.sourceId);
News.empty();
}
Now we shall modify our Main Dart Class to display news screen.
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Instant News',
theme: ThemeData(
// This is the theme of your application.
//
// Try running your application with "flutter run". You'll see the
// application has a blue toolbar. Then, without quitting the app, try
// changing the primarySwatch below to Colors.green and then invoke
// "hot reload" (press "r" in the console where you ran "flutter run",
// or simply save your changes to "hot reload" in a Flutter IDE).
// Notice that the counter didn't reset back to zero; the application
// is not restarted.
primarySwatch: Colors.blue,
backgroundColor: Color.fromRGBO(227, 244, 254, 1)),
home: MyHomePage(title: 'Instant News'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData.light(),
color: Color.fromRGBO(227, 244, 254, 1),
routes: <String, WidgetBuilder>{
"/":(context) => new NewsPage(),
},
);
}
_MyHomePageState() {
}
}
Then NewsPage class which has list of news, the list view is built using ListView.builder()
Class NewsPage extends StatelessWidget {
List<News> news;
NewsPage() {
news.add(News('title', 'content', 'description', 'https://blogs.ashrithgn.com/2019/06/20/set-up-app-loading-progress-indicator-dialog/', 'https://www.google.com/imgres?imgurl=https%3A%2F%2Fblogs.ashrithgn.com%2Fcontent%2Fimages%2F2019%2F06%2FSmartSelect_20190620-130838.gif&imgrefurl=https%3A%2F%2Fblogs.ashrithgn.com%2F2019%2F06%2F20%2Fset-up-app-loading-progress-indicator-dialog%2F&docid=0oPlF_qme663nM&tbnid=_D0UApTC5dGYLM%3A&vet=10ahUKEwiVle7FroLlAhVBSX0KHQ6tDyIQMwg7KAEwAQ..i&w=499&h=640&bih=626&biw=1366&q=flutter%20ashrith&ved=0ahUKEwiVle7FroLlAhVBSX0KHQ6tDyIQMwg7KAEwAQ&iact=mrc&uact=8',
null, 'ashrith', '1'));
news.add(News('title1', 'conten2t', 'description', 'https://blogs.ashrithgn.com/2019/06/20/set-up-app-loading-progress-indicator-dialog/', 'https://www.google.com/imgres?imgurl=https%3A%2F%2Fblogs.ashrithgn.com%2Fcontent%2Fimages%2F2019%2F06%2FSmartSelect_20190620-130838.gif&imgrefurl=https%3A%2F%2Fblogs.ashrithgn.com%2F2019%2F06%2F20%2Fset-up-app-loading-progress-indicator-dialog%2F&docid=0oPlF_qme663nM&tbnid=_D0UApTC5dGYLM%3A&vet=10ahUKEwiVle7FroLlAhVBSX0KHQ6tDyIQMwg7KAEwAQ..i&w=499&h=640&bih=626&biw=1366&q=flutter%20ashrith&ved=0ahUKEwiVle7FroLlAhVBSX0KHQ6tDyIQMwg7KAEwAQ&iact=mrc&uact=8',
null, 'ashrith', '1'));
}
@override
Widget build(BuildContext context) {
return ListView.builder(
itemBuilder: (BuildContext ctxt, int index) {
return _buildListItem(news[index], ctxt);
},
itemCount: news.length,
);
}
_buildListItem(News news, BuildContext context) {
return Card(
child: Container(
padding: EdgeInsets.only(top: 10),
child: Column(
children: <Widget>[
Container(
margin: EdgeInsets.only(top: 5, left: 15, bottom: 5, right: 5),
child: Text(
news.title == null || news.title.isEmpty
? "NA"
: news.title,
style:
TextStyle(fontWeight: FontWeight.bold, fontSize: 16))),
Container(
margin: EdgeInsets.only(bottom: 5, left: 25),
child: Text(
"Source: newsapi.org - ${news.sourceName}",
style: TextStyle(
fontWeight: FontWeight.normal,
color: Colors.grey,
fontSize: 10,
),
textAlign: TextAlign.left,
)),
Container(
margin: EdgeInsets.only(bottom: 15, top: 0),
decoration: BoxDecoration(color: Colors.grey),
child: news.imagePath == null || news.imagePath.isEmpty
? SizedBox(
height: 10,
)
: Image.network(news.imagePath, fit: BoxFit.fitWidth),
),
Container(
margin: EdgeInsets.only(top: 5, left: 10),
child: Text(
news.description == null || news.description.isEmpty
? "NA"
: news.description,
style: TextStyle(
fontWeight: FontWeight.normal, fontSize: 14))),
Divider(
height: 20,
color: Colors.grey,
),
Row(
children: <Widget>[
IconButton(
icon: Icon(Icons.remove_red_eye),
tooltip: "View",
onPressed: () async {
},
),
IconButton(
icon: Icon(Icons.share),
tooltip: "Share",
onPressed: () {
NativeShare.share({
'title': news.title == null || news.title.isEmpty
? "NA"
: news.title,
'url': news.path == null || news.path.isEmpty
? null
: news.path,
});
},
)
],
crossAxisAlignment: CrossAxisAlignment.end,
mainAxisAlignment: MainAxisAlignment.end,
)
],
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
),
),
margin: EdgeInsets.only(top: 10, left: 10, right: 10),
);
}
}
This example uses ListView.builder() as said in the title,using card layout to display the news,ListView.builder() is used when we have large number of list items which increases the the user experience by lazy loading the list items during scroll.
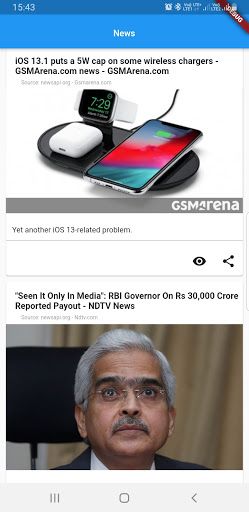