File upload in spring boot Rest Controller
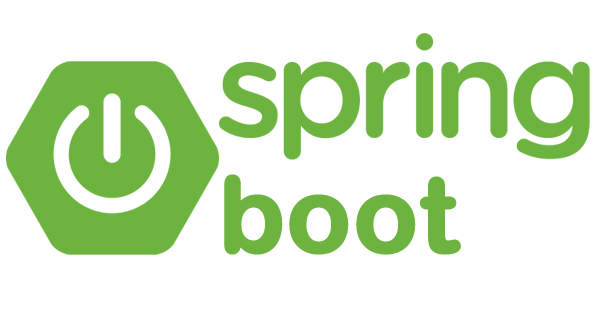
Spring boot is as an awesome framework where we could rapidly start our web development project with in minutes,java based configuration simplifies bootstrapping time where we could include third party library or other component into our applications. and to upload file in spring boot application is also made very simple just by configuring dependencies we are good to receive a file in our controller.so lets get started how to upload an file or files.
Step 1 : Adding required dependency.
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.1</version>
</dependency>
The Commons FileUpload bundle makes it simple to include vigorous, elite, document transfer capacity to your servlets and web applications.
FileUpload parses HTTP demands which comply with RFC 1867, "Structure based File Upload in HTML". That is, if a HTTP solicitation is submitted utilizing the POST strategy, and with a substance sort of "multipart/structure information", at that point FileUpload can parse that solicitation, and make the outcomes accessible in a way effectively utilized by the guest.
Step 2 : Controller function
@RequestMapping(path = "/", method = RequestMethod.POST, produces = MimeTypeUtils.APPLICATION_JSON_VALUE, consumes = {MediaType.MULTIPART_FORM_DATA_VALUE})
@ApiModelProperty(value = "key,value")
public POJOClass upload(
@RequestPart(value = "files", required = false) MultipartFile[] files, HttpServletRequest request) {
for (MultipartFile file : files) {
System.out.println(file.getOriginalFilename());
file.trasferTo(new File("/newpath.ext"))
}
return POJOCLASS
}
Things to remember:
MediaType.MULTIPART_FORM_DATA_VALUE
content type in the headers
@RequestPart
annotation in the request variable
MultipartFile
can be single file or array based on the requirement
trasferTo
to move from temporary location to desired location
``
Downloading File in Spring Boot
@RequestMapping(name = "file-download", path = "/v1/file-download",
method = RequestMethod.GET,
produces = MediaType.APPLICATION_JSON_VALUE)
public ResponseEntity<ByteArrayResource> fileDownload(HttpServletRequest request,
@RequestParam(value = "path", required = false) String path,
HttpServletResponse response
) {
try {
File temp = new File(path);
temp.canRead();
bytes[] res = Files.toByteArray(temp);
ByteArrayResource resource = new ByteArrayResource(data);
return ResponseEntity.ok()
.contentLength(res.length)
.header("Content-type", "application/octet-stream")
.header("Content-disposition", "attachment; filename=\"name.ext\"").body(resource);
}catch (IOException e) {
e.printStackTrace();
throw new RuntimeException("No such file or directory");
}
}