Ehcache: Java Based Cache in Spring Boot
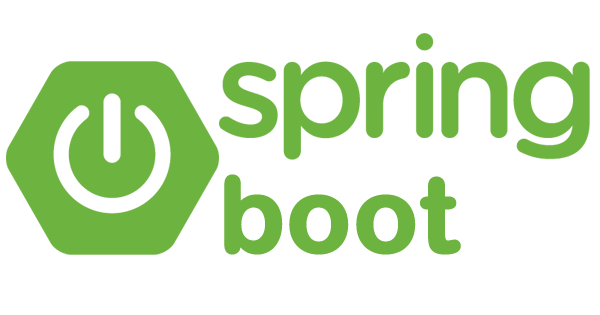
Most of the time, when we need to retrieve the same piece of data repeatedly from a data store, we generally try to cache the data to an in-memory cache. One such library we use when our data is lightweight is Ehcache. In this article, we shall see how to implement Ehcache in Spring boot by creating bean.
Step 1: Adding Dependency to 'pom.xml'
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.9.0</version>
</dependency>
Step 2: Creating the Bean Class to Creating Ehcache instance
@Configuration
public class EhCacheBean {
private Cache<String, String> ehCache;
@PostConstruct
public void init() {
CacheManager cacheManager = CacheManagerBuilder
.newCacheManagerBuilder()
.build();
cacheManager.init();
ehCache= cacheManager.createCache("applicationCache", CacheConfigurationBuilder
.newCacheConfigurationBuilder(
String.class, String.class,
ResourcePoolsBuilder.heap(100))
.withExpiry(ExpiryPolicyBuilder.timeToLiveExpiration(Duration.ofMinutes(50L))));
}
@Bean
Cache<String, String> getSpmCache() {
if (ehCache== null) {
init();
}
return ehCache;
}
}
Step3: Example to Add values to Cache and retrieve the Value from The cache.
At first Auto Wire the Cache instance in your service class like this
@Autowired
private Cache<String,String> appCache;
At next we can use the 'appCache' Variables to add the value like this
appCache.put(CACHE_KEY,VALUE);
And to retrieve
appCache.get(CACHE_KEY);
Finally to check if the key exist and remove the item we can use these
appCache.containsKey(CACHE_KEY)
appCache.remove(CACHE_KEY)