Difference between object and class in Scala.
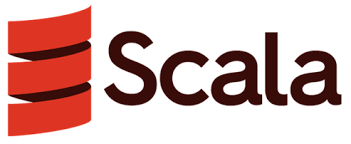
Object
so in scala object is a singleton instance of the class, lets explain with small snippet.
object test {
def addPlusOne(a:Int) = a+1;
}
So when your calling the function instance is already created you can just use like static functions of class in java i.e `test.addPlusOne(2)` so the object of `test` will have one instance through JVM. we can also use object as some special instance of Class and or traits like int the example below
class DummyMath {
def AdvanceSub(a:Int,b:Int,c:Int) = a+b+c;
}
trait Math {
def add(a:Int,b:Int):Int = a+b;
def sub(a:Int,b:Int):Int = a-b;
}
trait AdvanceMath {
def advanceAdd(a:Int,b:Int,c:Int) = a+b+c
}
object MathHandler extends DummyMath with AdvanceMath with Math {
}
so in our main function without creating the instance of DummyMath
just by extending we can call the methods of dummyMath and other functions of Traits like this
object Main extends App {
MathHandler.add(1,2);
MathHandler.advanceAdd(1,2,3);
MathHandler.AdvanceSub(1,2,3)
}
Class
just like any other object oriented language we need to create instance manually and call the methods inside the class. so to understand better we shall use the sample snippet
class test {
def addPlusOne(a:Int) = a+1;
}
to use the above class we need to create instance with new keyword new Test().addPlusOne(1);