Getting Started and Deploying Spring Boot application on Google App Engine (GAE)
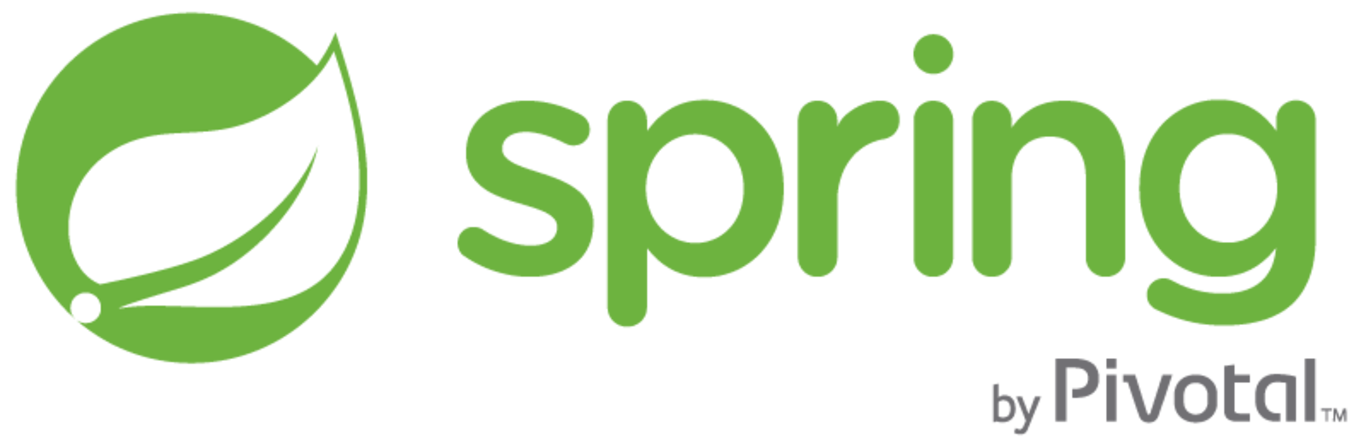
So spring boot is versatile web framework and pretty much every java developer these days use this and Google app engine or GAE is a Platform as a Service and cloud computing platform for developing and hosting web applications.
So using GAE will eliminate infrastructure management by auto scaling when required, these days also called as Server less architecture, internally google will use kubernetes containers and scale based on usage, GAE supports python, java, node,php as far as i know. now lets dive into creating spring boot application and deploying it.
Before this tutorial you need valid account on google cloud platform, and application created on GAE (google app engine), and google cli installed on your system.
- lets create spring boot app, and effective pom will look like this
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.8.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.ashrithgn.appengine</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>demo</name>
<packaging>war</packaging>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
<spring-cloud.version>Greenwich.SR3</spring-cloud.version>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-gcp-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- add following dependency under dependencies section -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jetty</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-gcp-starter</artifactId>
<version>1.1.3.RELEASE</version>
<type>pom</type>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
<plugin>
<groupId>com.google.cloud.tools</groupId>
<artifactId>appengine-maven-plugin</artifactId>
<version>2.1.0</version>
<configuration>
<deploy.projectId>project-id</deploy.projectId>
<deploy.version>1</deploy.version>
</configuration>
</plugin>
</plugins>
</build>
</project>
- Create appengine-web.xml in the path
<project>/main/webapp/WEB-INF/appengine.xml
with following content
<?xml version="1.0" encoding="UTF-8"?>
<appengine-web-app xmlns="http://appengine.google.com/ns/1.0">
<application><your app id></application>
<version>3</version>
<threadsafe>true</threadsafe>
<runtime>java8</runtime>
</appengine-web-app>
- Create app.yaml in the path
<project>/main/appengine/app.yaml
with following content
runtime: java
env: flex
runtime_config: # Optional
jdk: openjdk8
handlers:
- url: /.*
script: this field is required, but ignored
manual_scaling:
instances: 1
- Override Application.java or main class to use external serverlet example code
@SpringBootApplication
public class DemoApplication extends SpringBootServletInitializer {
@Override
protected SpringApplicationBuilder configure(SpringApplicationBuilder application) {
return application.sources(DemoApplication.class);
}
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
- Sample rest controller example
@RestController
public class Controller {
@GetMapping(path = "/test")
public String hello() {
return "i am working bro";
}
}
- To test mvn appengine:run
- To deploy mvn appengine:run