Custom Annotation To Handle Authorisation In Spring Boot AOP Tutorial
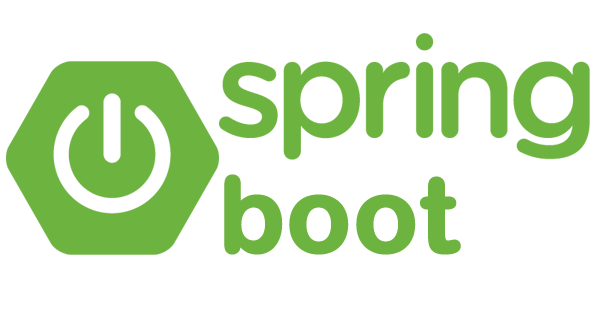
Overview:
- Creating custom annotation
- Creating component in spring boot
- Creation a configuration and middleware in spring boot
- Basic of Aspect and usage in spring boot
What is Aspect In Spring Boot ?
Aspects are cross cutting concerns like logging,security in different layers for the ease of code maintenance .
Key Dependency required
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
<version>5.0.1.RELEASE</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.8.12</version>
<scope>compile</scope>
</dependency>
- Spring AOP provides basic AOP Capabilities.
- AspectJ provides a complete AOP framework.
-
STEP 1 : Lets create an annotation
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD) // can use in method only.
public @interface Authorized {
public boolean enabled() default true;
}
@Retention(RetentionPolicy.RUNTIME) : instruct compiler to retain the annotation during runtime
@Target(ElementType.METHOD) : this suggest where this annotation can be used
-
Step 2 : Lets Create a file which actually implement logic of authorization
@Component
public class AuthorizationImpl {
public boolean authorize(String token) {
// implemnt jwt or any any token based authorization logic
return true;
}
}
-
Step 3 Aspect file which acts as middleware which intercept the the request and authorize and proceed
@Aspect
@Configuration
public class AuthAspect {
@Autowired
AuthorizationImpl authBean;
@Before("@annotation(com.<packagepath>.Authorized) && args(request,..)")
public static void before(HttpServletRequest request){
if (!(request instanceof HttpServletRequest)) {
throw
new RuntimeException("request should be HttpServletRequesttype");
}
if(authBean.authorize(request.getHeadr("Authorization"))){
req.setAttribute(
"userSession",
"session information which cann be acces in controller"
);
}else {
throw new RuntimeException("auth error..!!!");
}
}
}
-
Step 3 : Usage of the annotation to authorize the request
@RestController
@RequestMapping(path = "/activities-to-jobs",
produces = {APPLICATION_JSON_VALUE},
headers = {"Authorization"})
public class XxxxController {
@RequestMapping(path = "/{id}/note", method = RequestMethod.GET)
@Authorized
public opDTO getSomeResult(HttpServletRequest request,....){
}
}