AWS S3 & Micronaut: File upload and download using Java
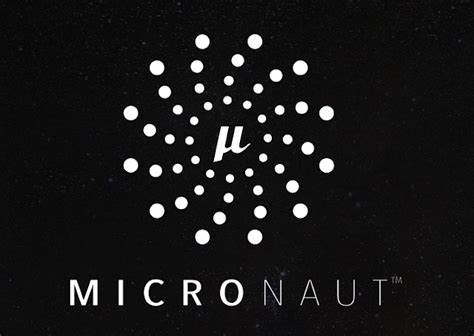
I started learning new framework called Micronaut, Micronaut is lightweight Java web framework, Which is cloud native in nature which support Java, Groovy and Kotlin and main advantage of the micronut its light weight, has low memory footprint and fast, one of the way it achieve its Fast nature and utilize low memory is by eliminating Java reflections.
Every one At least heard of AWS, And if your developer with bit of experience you might have used AWS services. So AWS S3 is called as "Simple Storage Solution" is provided as a Service on AWS platform, And This is Object based store. Instead of storing a file traditionally on File store, it saves as an Object, Which enables lot of benefits, like part of file can replicated, can be broken as multiple parts and could be stored on different physical location. Now We shall Dive into the coding part.
To Setup file upload in Micronut Follow this:
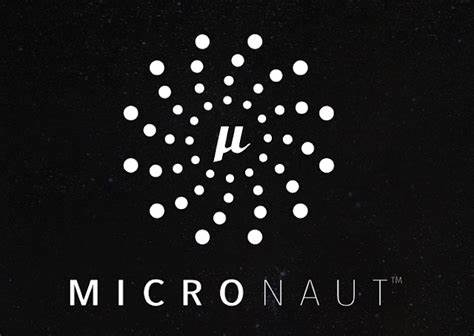
Add the AWS S3 Maven Dependency:
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>s3</artifactId>
<version>2.16.22</version>
</dependency>
Creating a Connection to AWS S3 Bucket.
To create there are few prerequisite like AWS application Key, AWS Secret key, And Empty bucket created with write access for the application key. so now we shall proceed with creating configuration with provides Amazon's S3Client Instance.
@Singleton
public class S3SBean {
@Inject
Environment env;
@Bean
public S3Client getS3Client() {
AwsBasicCredentials credentials = AwsBasicCredentials.create(env.get("aws.clientId",String.class)),
env.get("aws.clientSecret", String.class"));
S3Client client = S3Client.builder().region(Region.US_EAST_1)
.credentialsProvider(StaticCredentialsProvider.create(credentials)).build();
return client;
}
}
Class Which Handles the Upload and download Files from the AWS S3.
I name this class as adapter, this implements the S3's function for our requirement, This works as wrapper for the S3 client, We could also name this as Wrapper.
@Singleton
public class S3Adapter {
@Inject
S3SBean amazonS3Client;
@Value("${s3.buckek.name}")
String defaultBucketName;
@Value("${s3.default.folder}")
String defaultBaseFolder;
public List<Bucket> getAllBuckets() {
return amazonS3Client.getS3Client().listBuckets().buckets();
}
public void uploadFile(File uploadFile) {
PutObjectRequest request = PutObjectRequest.builder()
.bucket(defaultBucketName)
.key(defaultBaseFolder+"/"+uploadFile.getName()).build();
amazonS3Client.getS3Client().putObject(request,uploadFile.toPath());
}
public void uploadFile(String fileName,byte[] fileBytes) {
File file = new File("../"+fileName);
file.canWrite();
file.canRead();
FileOutputStream iofs = null;
try {
iofs = new FileOutputStream(file);
iofs.write(fileBytes);
PutObjectRequest request = PutObjectRequest.builder()
.bucket(defaultBucketName)
.key(defaultBaseFolder+"/"+file.getName()).build();
amazonS3Client.getS3Client().putObject(request,file.toPath());
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public byte[] getFile(String key) {
GetObjectRequest getObjectRequest = GetObjectRequest.builder()
.bucket(defaultBucketName).key(defaultBaseFolder+"/"+key).build();
ResponseInputStream<GetObjectResponse> obj = amazonS3Client.getS3Client().getObject(getObjectRequest);
try {
byte[] content = IOUtils.toByteArray(obj);
return content;
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
}
Writing Rest API to upload and download files through and fro from AWS S3 buckets.
@Controller
public class FileController {
@Inject
S3Adapter s3Adapter;
@Post(value = "/upload", consumes = {MediaType.MULTIPART_FORM_DATA})
public boolean uploadFile(@Part CompletedFileUpload file) throws IOException {
s3Adapter.uploadFile(file.getFilename(),file.getBytes());
return true;
}
@Get(value = "/download{?fileName}", consumes = {MediaType.MULTIPART_FORM_DATA})
public HttpResponse<byte[]> downLoadFile(@Nullable String fileName) throws IOException {
return HttpResponse.ok(s3Adapter.getFile(fileName))
.header("Content-type", "application/octet-stream")
.header("Content-disposition", "attachment; filename=\""+fileName+"\"");
}
}
So now now open the API testing tool and call the API to check weather the code is working :-P, basically there are API to list bucket, upload the file and download the file.
Main Class of the Micronut's Application
public class Application {
public static void main(String ...args) {
Micronaut.run(args);
}
}
Same Article on Spring boot with legacy dependency
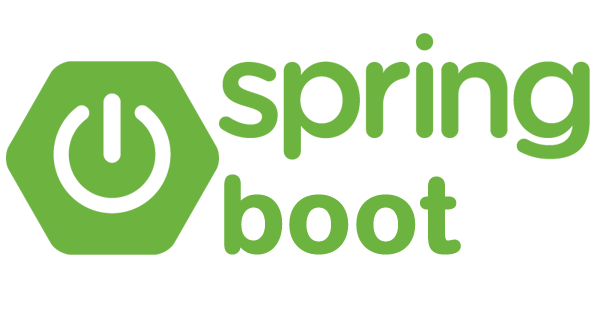